Event listeners in turtle graphic is a great way to engage an user to do something on the turtle screen. In today's lesson, we learned about Event Listeners, Higher Order Functions, State & instances in python.
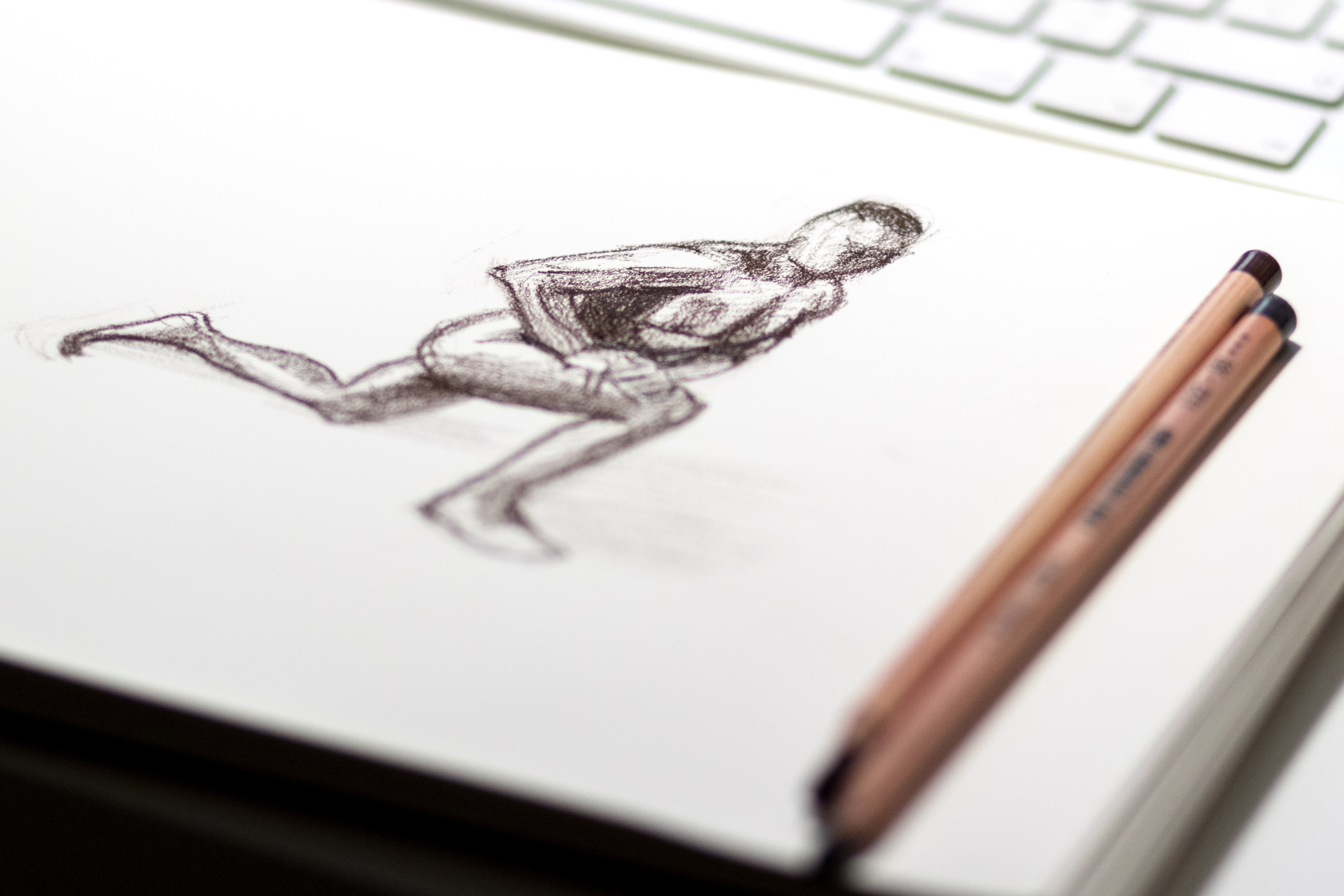
The first part of today's lesson had an exercise to make an Etch a Sketch game where an user can use several keys to move the turtle to draw. In this project, we learned how to use higher order function. A higher order function is a function that takes another function as an arguement. By using the screen.onkey() method, I've created the movements of the turtle. While working though this project, I've also gave thought about using the turtle.onkeypress() function, and so, I've created another brunch on github just for this experiment. AND IT WORKED!!! 😅 Here's the code snippet -
from turtle import Turtle, Screen
# Creating necessary objects
tim = Turtle()
screen = Screen()
tim.speed("fastest")
# Functions for the movement
def move_fd():
tim.forward(10)
def move_bk():
tim.backward(10)
def move_cw():
tim.right(10)
def move_ccw():
tim.left(10)
def world_clear():
tim.clear()
tim.penup()
tim.home()
tim.pendown()
# Call the listen method for Screen
screen.listen()
# Call the onkey method for the movements of turtle
screen.onkey(key="w", fun=move_fd)
screen.onkey(key="s", fun=move_bk)
screen.onkey(key="a", fun=move_cw)
screen.onkey(key="d", fun=move_ccw)
screen.onkey(key="c", fun=world_clear)
# Screen exit method
screen.exitonclick()
The full project can be accessed via my github repository. There is another branch where I've used the continuous drawing method with the help of turtle.onkeypress() method. With this and more, I'll post tomorrow again in sha Allah and will share what I'll learn tomorrow.